7 Programming Paradigms that Present Challenges and How to Overcome Them
IT Professors

7 Programming Paradigms that Present Challenges and How to Overcome Them
Delving into the complexities of various programming paradigms presents a unique set of challenges. This article draws on expert insights to navigate these intricacies, offering practical solutions for mastering even the most daunting programming techniques. Explore the depths of functional, concurrent, and event-driven programming, among others, and learn how to effectively implement these paradigms in your own projects.
- Functional Programming: Challenges and Solutions
- Embracing Functional Concepts Through Practice
- Mastering Concurrent Programming Techniques
- Logic Programming: A Declarative Approach
- Aspect-Oriented Programming Enhances Modularity
- Event-Driven Programming Creates Responsive Apps
- Metaprogramming: Generating Code Dynamically
Functional Programming: Challenges and Solutions
One programming paradigm I find particularly challenging is functional programming (FP), especially when transitioning from an object-oriented (OOP) mindset. The emphasis on immutability, pure functions, and recursion instead of traditional loops requires a fundamental shift in how problems are approached. Unlike OOP, where state and behavior are encapsulated in objects, FP encourages stateless computations, which can be difficult to manage when dealing with complex, large-scale data transformations. Additionally, concepts like higher-order functions, monads, and category theory in languages like Haskell and Scala can be mathematically abstract, making them harder to grasp for developers accustomed to imperative programming.
One of the biggest challenges is managing immutability efficiently. In FP, modifying data structures is discouraged, which can create performance concerns when dealing with high-volume data processing. Instead of mutating values, developers must use persistent data structures or recursion, which can be unintuitive compared to traditional loops. Another challenge is understanding function composition and monads, which require a more mathematical approach to programming.
To overcome these challenges, I take a practical, hands-on approach by writing small FP programs in Python using map, reduce, and functools, which help reinforce key concepts. I also refactor OOP-based solutions into FP, focusing on pure functions, immutability, and composability to gradually apply functional principles. Additionally, I leverage hybrid paradigms in languages like JavaScript, Kotlin, and Scala, where I can mix functional and object-oriented approaches, allowing for a smoother transition.
By breaking down FP concepts into real-world applications, I've significantly improved my ability to write scalable, maintainable, and parallelizable code. While FP can be difficult to master, its ability to simplify concurrent programming, reduce side effects, and improve testability makes it an essential skill for modern software development.
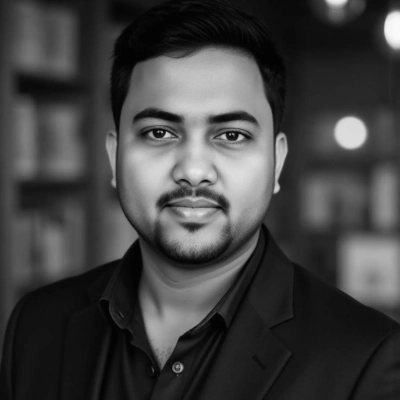
Embracing Functional Concepts Through Practice
I find the functional programming paradigm particularly challenging due to its emphasis on immutability, higher-order functions, and often abstract concepts like monads and pure functions. Coming from a more familiar imperative and object-oriented background, it takes a significant mindset shift to embrace stateless design and recursion as primary tools for problem-solving. The declarative nature of functional programming means that the logic is expressed in a very different way, which can initially feel unintuitive and requires a deep understanding of mathematical concepts to fully grasp.
To overcome these challenges, I immerse myself in focused study and practical exercises, starting with foundational texts like "Learn You a Haskell for Great Good!" and working through coding challenges on platforms like Exercism or Codewars. I also find it helpful to participate in study groups and pair programming sessions with colleagues experienced in functional programming, which accelerates my learning through collaborative problem-solving and code reviews. This combination of theoretical learning, hands-on practice, and peer support has significantly improved my ability to think functionally and integrate those concepts into my work.
Mastering Concurrent Programming Techniques
Concurrent programming presents challenges due to its complex nature of handling multiple tasks simultaneously. Developers often struggle with race conditions, deadlocks, and synchronization issues. To overcome these hurdles, it's crucial to utilize synchronization primitives such as mutexes, semaphores, and locks effectively. Additionally, implementing well-established design patterns like the producer-consumer pattern or the reader-writer pattern can greatly simplify concurrent code.
Practice and patience are key when working with concurrent programming, as it requires a different mindset compared to sequential programming. Start with small concurrent projects and gradually increase complexity as skills improve. Embrace the challenge and dive into concurrent programming to enhance your software development capabilities.
Logic Programming: A Declarative Approach
Logic programming, while powerful for certain problem domains, can be difficult for developers accustomed to imperative or object-oriented paradigms. This approach focuses on describing facts and relationships rather than specifying step-by-step instructions. To overcome the initial learning curve, beginners should start with simple rule-based systems and gradually build up to more complex logic programs. Understanding concepts like unification and backtracking is crucial for mastering logic programming.
Prolog, a popular logic programming language, offers an excellent starting point for exploring this paradigm. As skills improve, tackle increasingly complex problems that showcase the strengths of logic programming. Embrace the declarative nature of logic programming and unlock its potential for solving complex reasoning tasks.
Aspect-Oriented Programming Enhances Modularity
Aspect-oriented programming (AOP) introduces a new way of thinking about program structure, which can be challenging for developers used to traditional paradigms. AOP aims to increase modularity by separating cross-cutting concerns, but implementing it effectively requires careful planning and design. To overcome the learning curve, start by identifying common cross-cutting concerns in existing projects, such as logging or security. Gradually introduce AOP concepts and tools, such as AspectJ for Java, to address these concerns separately from the main business logic.
It's important to maintain a balance between using aspects and traditional object-oriented design. As proficiency grows, explore more advanced AOP techniques and their applications in large-scale software systems. Dive into aspect-oriented programming to enhance code modularity and maintainability in your projects.
Event-Driven Programming Creates Responsive Apps
Event-driven programming can be challenging due to its non-linear flow and the need to manage complex event interactions. This paradigm requires a shift in thinking from sequential execution to responding to various events and triggers. To master event-driven programming, start by practicing with small reactive applications that handle user inputs or system events. Understanding concepts like event loops, callbacks, and event emitters is crucial for success in this paradigm.
As skills improve, explore more complex event-driven architectures and frameworks popular in web and mobile development. It's important to develop strategies for managing event-related state and avoiding callback hell. Embrace the event-driven approach to create more responsive and interactive applications that can handle real-time updates efficiently.
Metaprogramming: Generating Code Dynamically
Metaprogramming, the practice of writing code that generates or manipulates other code, can be intimidating due to its abstract nature and potential complexity. This paradigm requires a deep understanding of the language's internals and reflection capabilities. To overcome the challenges of metaprogramming, begin by exploring code generation techniques and simple macro systems. Gradually progress to more advanced topics like abstract syntax trees and runtime code modification.
It's crucial to maintain a balance between the power of metaprogramming and code readability and maintainability. As skills improve, explore how metaprogramming can be used to reduce boilerplate code and create more flexible and extensible software systems. Dive into the world of metaprogramming to unlock new levels of abstraction and code reuse in your projects.